Variables in Bash
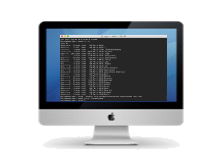
Using variables in Bash is easy to learn if you're familiar with PHP programming.
Four Simple tips when using variables in Bash
- Keep it simple
- Use variable parameters to expand the script capabilities
- Watch for extra spacing when using the command expansion ${
- When using characters as values make sure to use double quotes.
Learn by Example
One of the first Web programming books that I found useful was "Perl by Example." It's a great book to learn various Perl functionality. ( I still have the book!)
I got one of the early editions which was a much smaller book and a lot easier to understand
So here are some Variable examples in BASH:
Simple HelloWorld.sh Example
Basics of assigning a text value to a BASH variable:
#!/bin/bash
STR="Hello World!"
echo $STR
BASH script with Variable Parameters
You can pass additional parameters to you BASH script at run time:
#!/bin/sh
#
# Echo a couple of script input values
# usage:
# name
#
# The result will be an echo of the first and last
if [ $# != 3 ]; then
echo "usage: name.sh first middle last"
exit 0
fi
FIRST="$1"
MIDDLE="$2"
LAST="$3"
echo $FIRST . " " . $LAST
Execute Command Lines via BASH variables
You can assign the results of an command line to a BASH variable
#!/bin/bash
# Get the last day of March
lastday=$(date -v+3w -v1d -v+1m -v-1d +"%B %e, %Y")
echo $lastday
Simple Math in Bash
#!/bin/bash
num1=2017
num2=1975
total=$(($num1 - $num2))
echo "Did you know 1975 was $total years ago!"
Miscellaneous Notes
In Bash $$ is the process ID, as noted in the comments it is not safe to use as a temp filename for a variety of reasons.